If you do not want to use any of the animation libraries, you can use your own animations, created using CSS3 animations properties. But you must not use css positions properties (Position, Top, Left, Right, Bottom).
jQuery Carousel
A lightweight and responsive Carousel. Which uses CSS3 Animations to change slide.
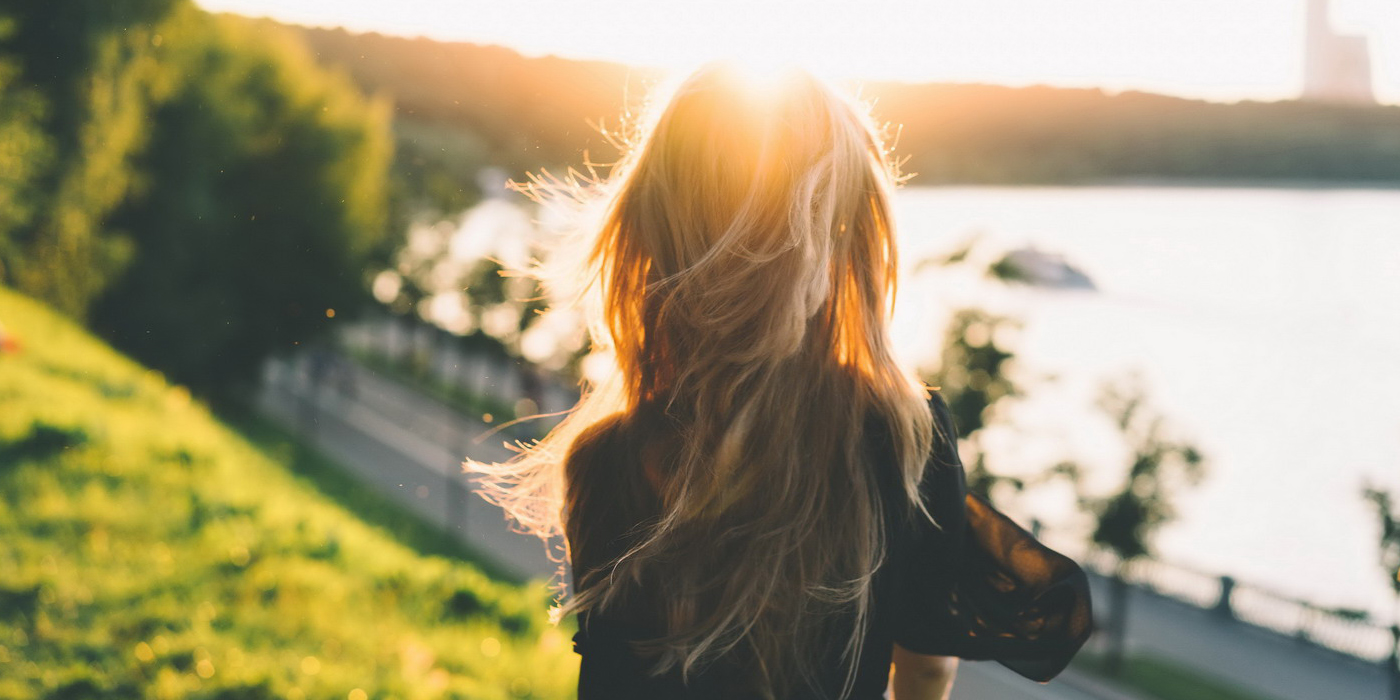
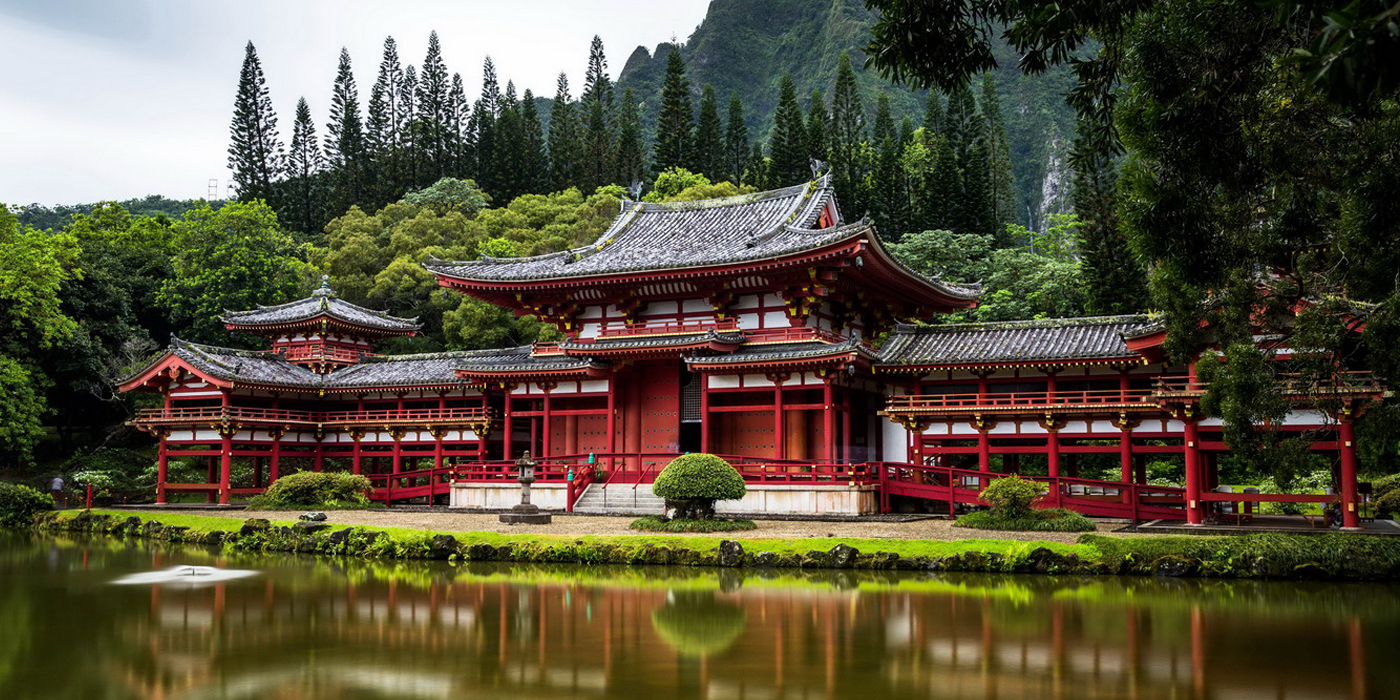
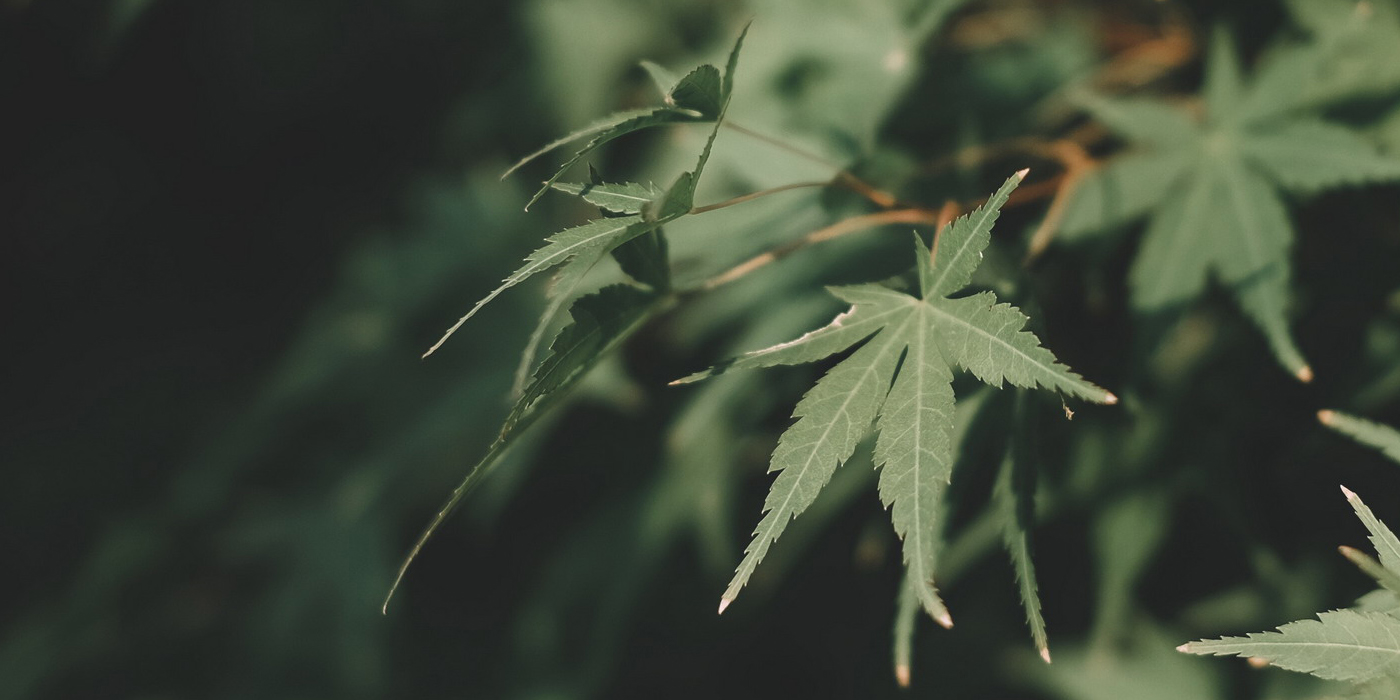
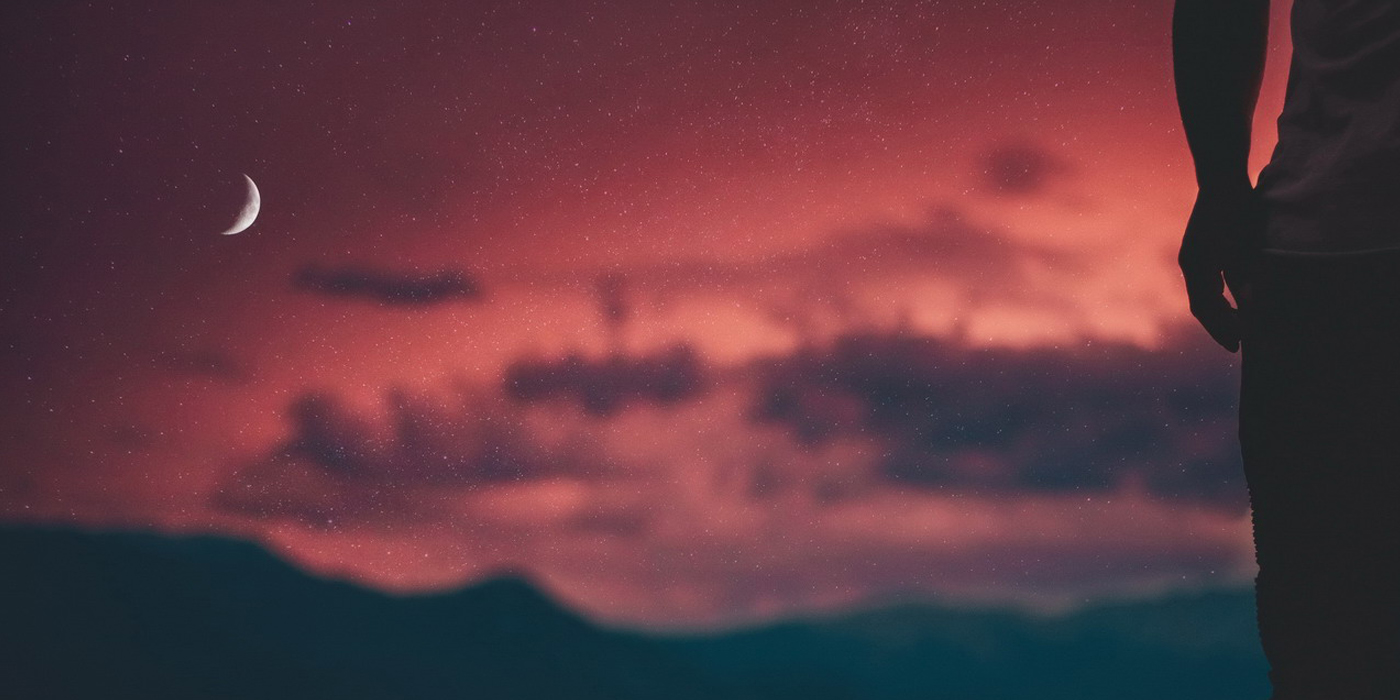
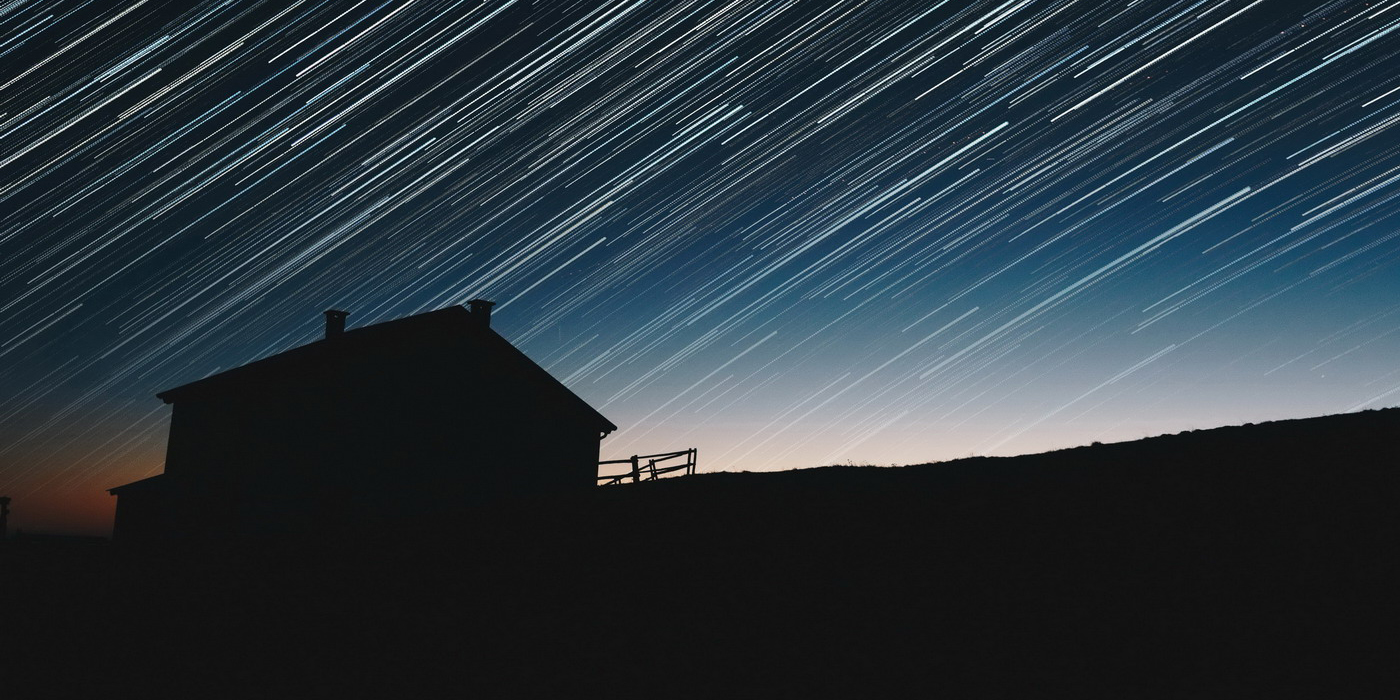
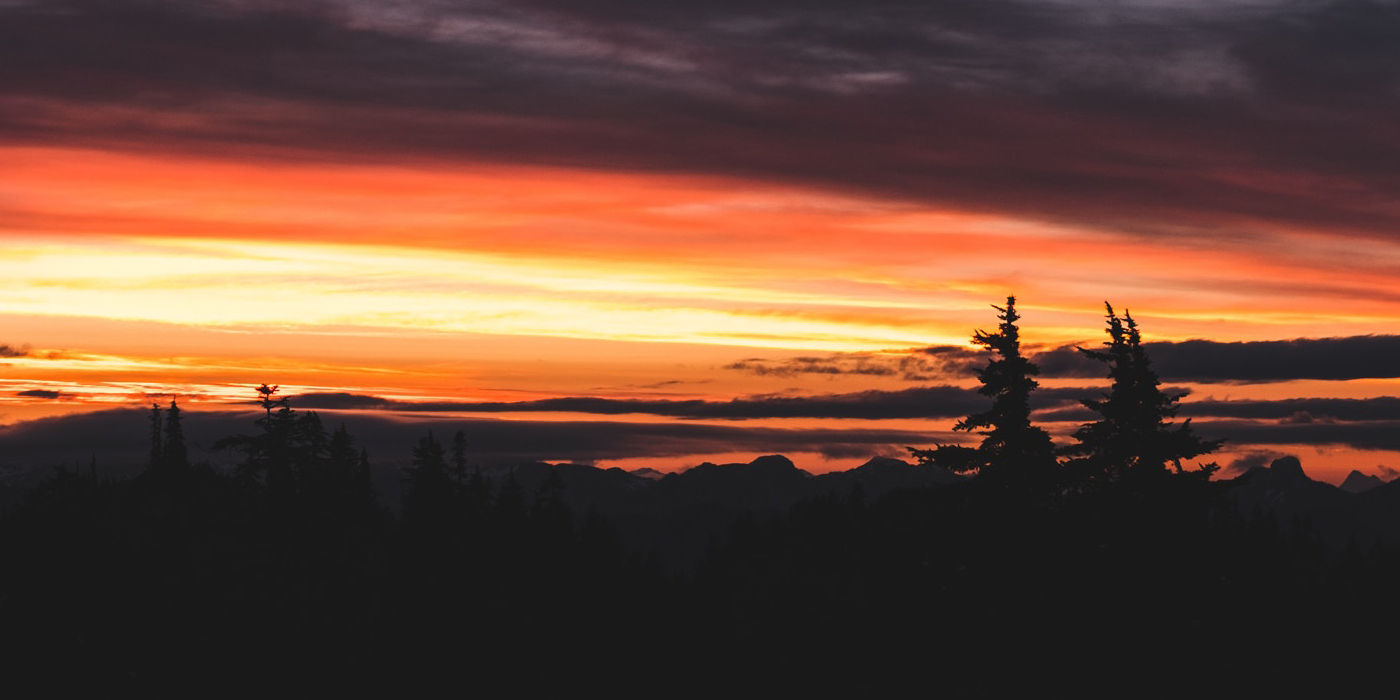
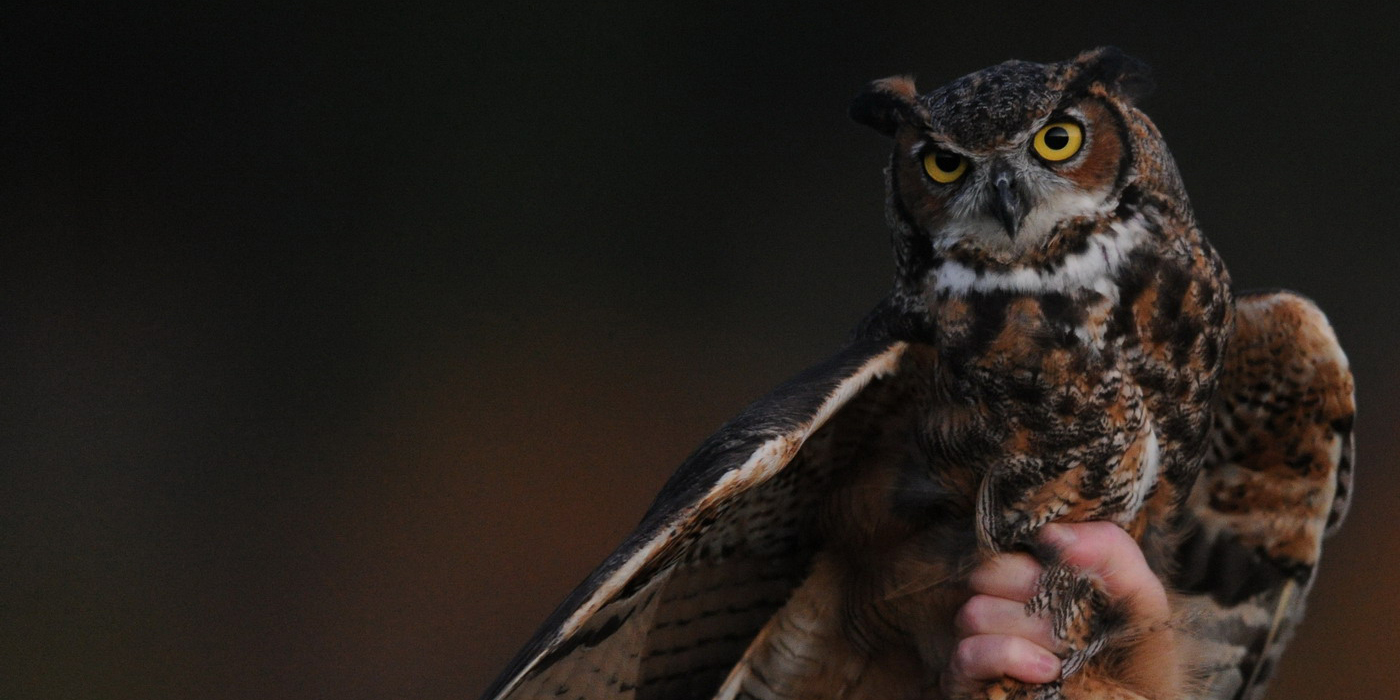
Getting Started
Requirements
There are a couple of requirements you need to meet before you can use jQuery Animate.css Carousel, those are jQuery library, and 1 of the CSS animation libraries listed below:
Installation
Installing jQuery Animate.css Carousel is pretty straight forward. Simply include the jQuery, CSS Animation library (eg. Animate.css), jQuery Animate.css Carousel's JavaScript and CSS file, like so:
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/animate.css/3.5.2/animate.min.css" type="text/css" media="screen" />
<link rel="stylesheet" href="css/animatecss-carousel.css" type="text/css" media="screen" />
<script type="text/javascript" src="https://ajax.googleapis.com/ajax/libs/jquery/1.10.2/jquery.min.js"></script>
<script type="text/javascript" src="js/animatecss-carousel.js"></script>
Notice the jQuery library I included was google code library, We recommend using latest version.
It is highly recommended that all JavaScript be placed after all your content at the footer of the page, just before the end </body>
tag. This ensures that all content is loaded before manipulation of the DOM occurs.
Usage
Basic
Create a
<div>
with an ID or Class. And inside of it create seperate <div>
for every slides/items.
<!-- HTML -->
<div id="aniCarousel-basic-use">
<div>
<img style="display:block; width:100%; margin:auto;" src="https://webbymage.com/wp-content/uploads/2020/05/plugin-carousel-horizontal-1.jpg" />
</div>
<div>
<img style="display:block; width:100%; margin:auto;" src="https://webbymage.com/wp-content/uploads/2020/05/plugin-carousel-horizontal-2.jpg" />
</div>
<div>
<img style="display:block; width:100%; margin:auto;" src="https://webbymage.com/wp-content/uploads/2020/05/plugin-carousel-horizontal-3.jpg" />
</div>
<div>
<img style="display:block; width:100%; margin:auto;" src="https://webbymage.com/wp-content/uploads/2020/05/plugin-carousel-horizontal-4.jpg" />
</div>
<div>
<img style="display:block; width:100%; margin:auto;" src="https://webbymage.com/wp-content/uploads/2020/05/plugin-carousel-horizontal-5.jpg" />
</div>
</div>
Now activate the jQuery Animate.css Carousel like this
$('#aniCarousel-basic-use').aniCarousel();
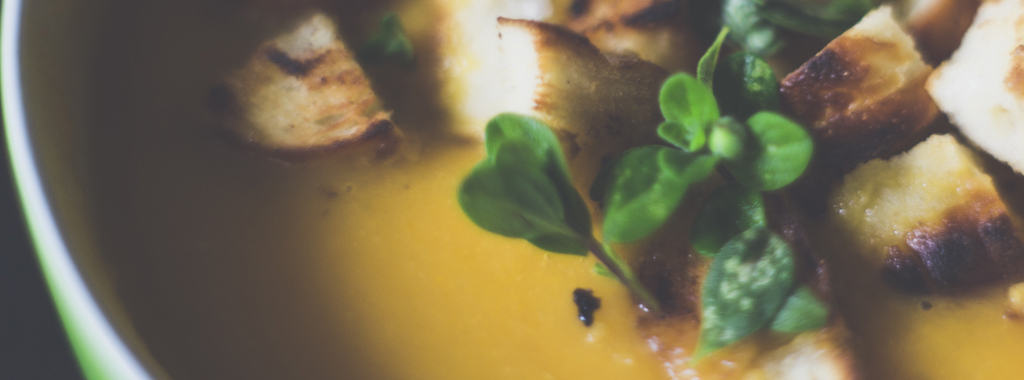
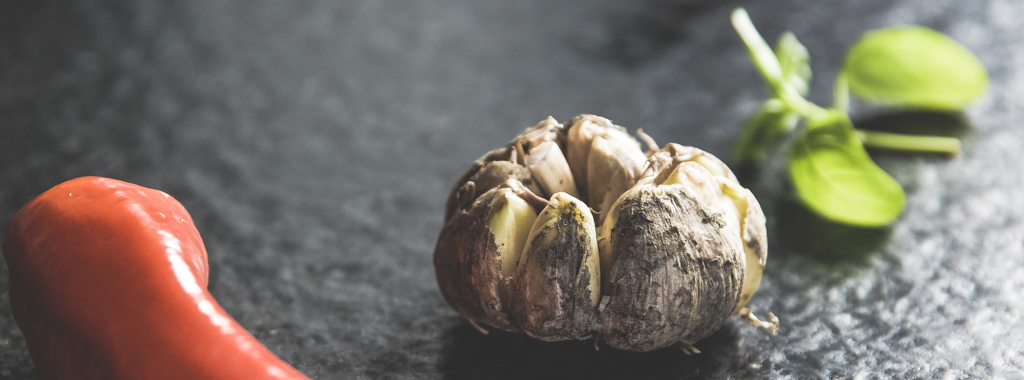
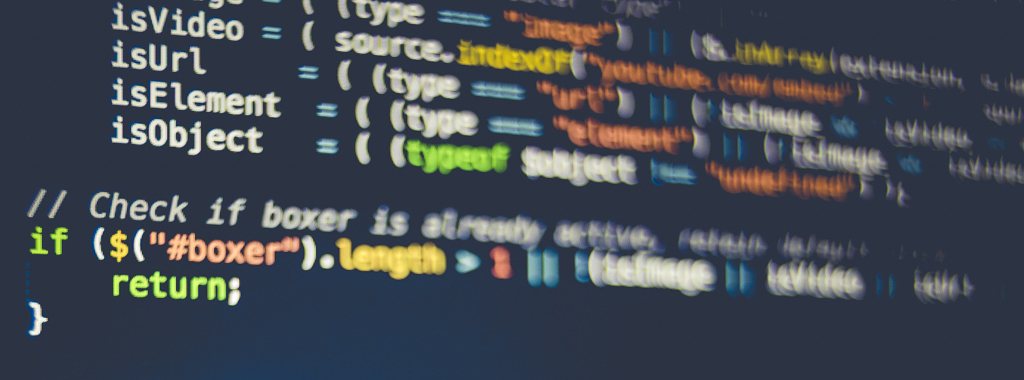
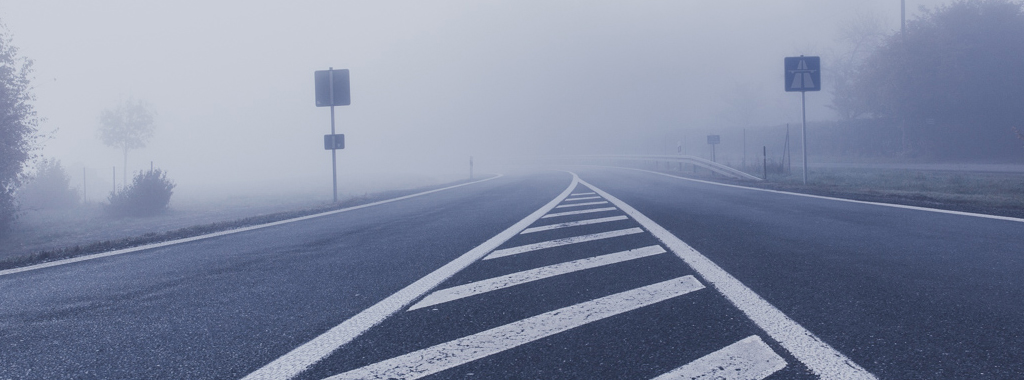
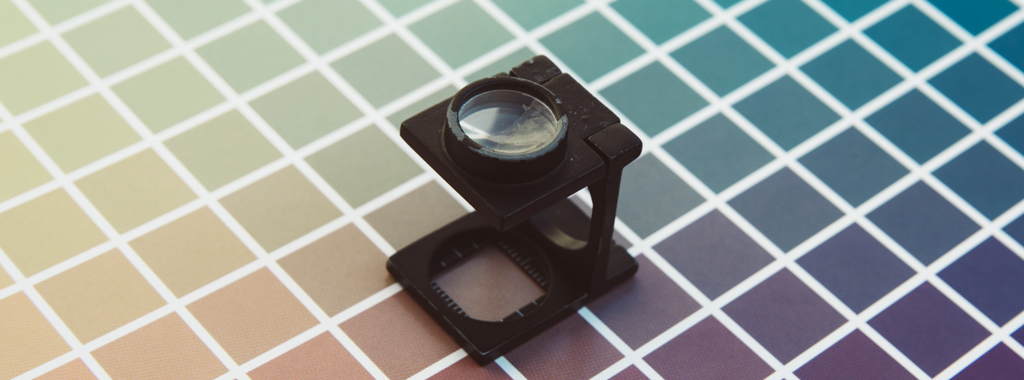
With options
Another example with some options defined.
$('#aniCarousel-with-options').aniCarousel({
animator: 'tuesday',
animationIn: 'tdStampIn',
animationOut: 'tdSwingOut',
loop: true,
overflow: 'hidden',
interval: 10,
paginator: true,
pauseOnHover: true
});
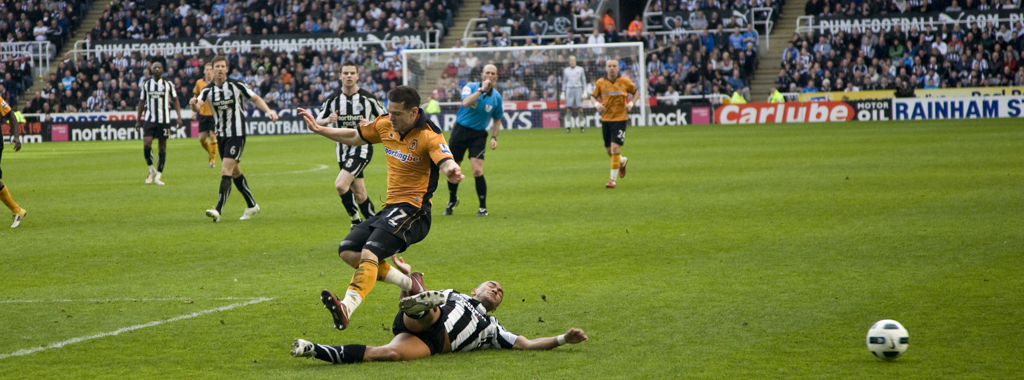
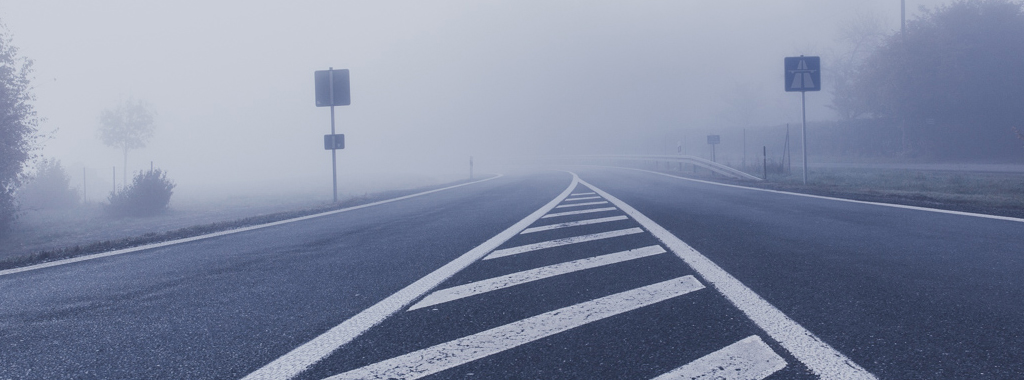
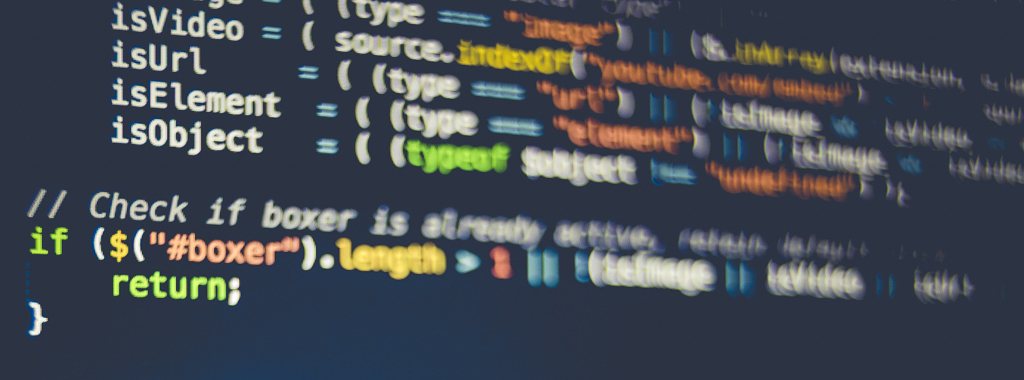
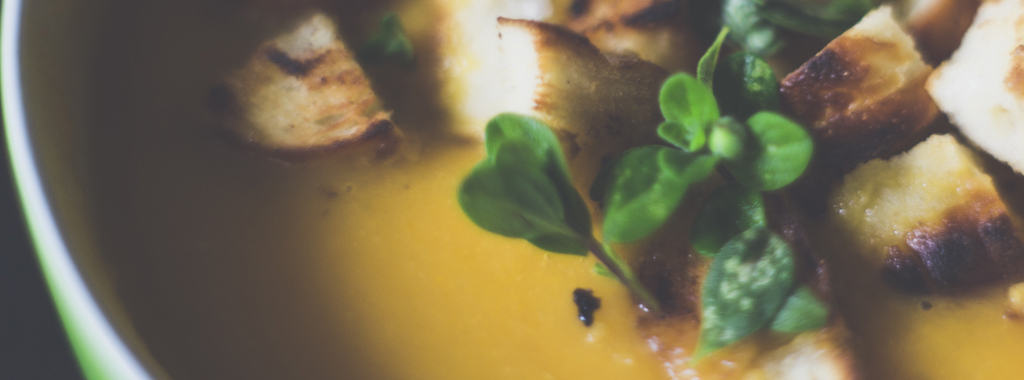
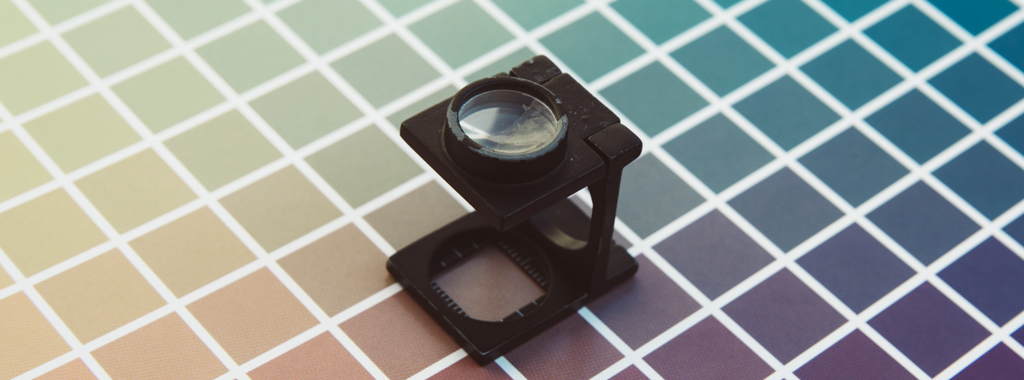
With Data Attributes
3 Special features of this Carousel is that you can give unique Animations for In and Out. And, You can also deifine duration for each slide. To do these, jQuery Animate.css Carousel give you 3 data attributes.
data-acc-in
- In animation for the slide/item.data-acc-out
- Out animation for the slide/item.data-acc-duration
- Duration (in seconds) of how long the slide will show whenautoplay
is set to 'true'.
<!-- HTML -->
<div id="aniCarousel-with-dataattributes">
<div data-acc-in="fadeIn" data-acc-out="fadeOut" data-acc-duration="5" >
<img style="display:block; width:100%; margin:auto;" src="https://webbymage.com/wp-content/uploads/2020/05/plugin-carousel-horizontal-1.jpg" />
</div>
<div data-acc-in="bounceIn" data-acc-out="bounceOut" data-acc-duration="6" >
<img style="display:block; width:100%; margin:auto;" src="https://webbymage.com/wp-content/uploads/2020/05/plugin-carousel-horizontal-2.jpg" />
</div>
<div data-acc-in="flipInX" data-acc-out="flipOutX" > <!-- data-acc-duration isn't defined. It will be using 'interval' from Options -->
<img style="display:block; width:100%; margin:auto;" src="https://webbymage.com/wp-content/uploads/2020/05/plugin-carousel-horizontal-3.jpg" />
</div>
<div data-acc-in="lightSpeedIn" > <!-- data-acc-out isn't defined. It will be using 'animationOut' from Options -->
<img style="display:block; width:100%; margin:auto;" src="https://webbymage.com/wp-content/uploads/2020/05/plugin-carousel-horizontal-4.jpg" />
</div>
<div data-acc-out="lightSpeedOut" > <!-- data-acc-in isn't defined. It will be using 'animationIn' from Options -->
<img style="display:block; width:100%; margin:auto;" src="https://webbymage.com/wp-content/uploads/2020/05/plugin-carousel-horizontal-5.jpg" />
</div>
</div>
Note: You might still want to define animationIn
, animationOut
and interval
, so if you do not define any of the Data attributes, script will take values from these 3 options.
$('#aniCarousel-with-dataattributes').aniCarousel({
animationIn: "zoomIn",
animationOut: "zoomOut",
interval: 5,
loop: true,
overflow: 'hidden',
autoplay:true,
paginator: true,
pauseOnHover: true,
});
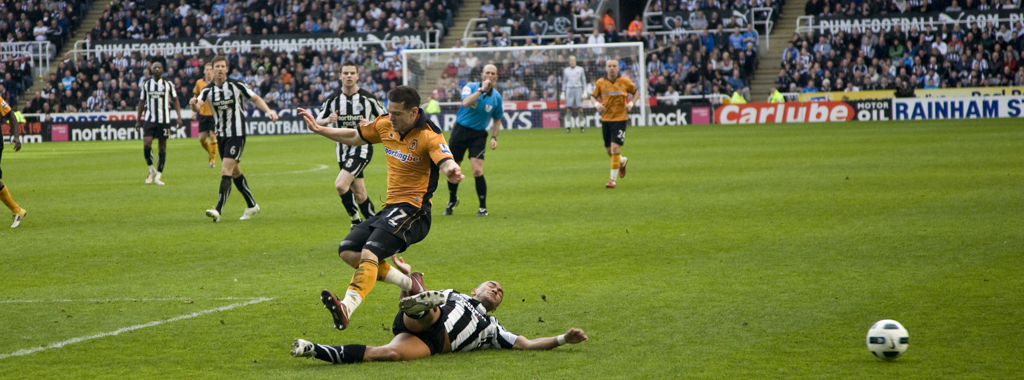
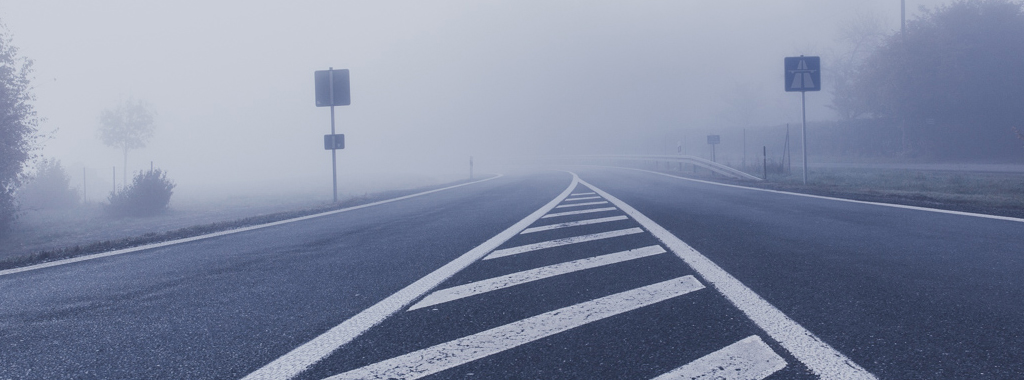
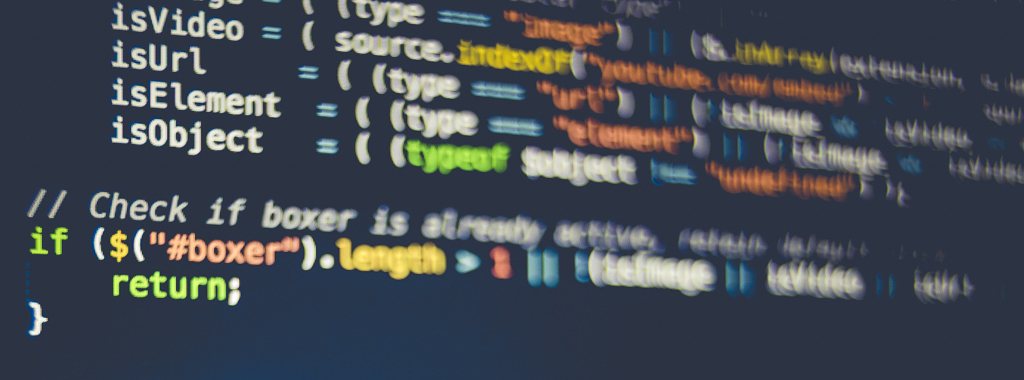
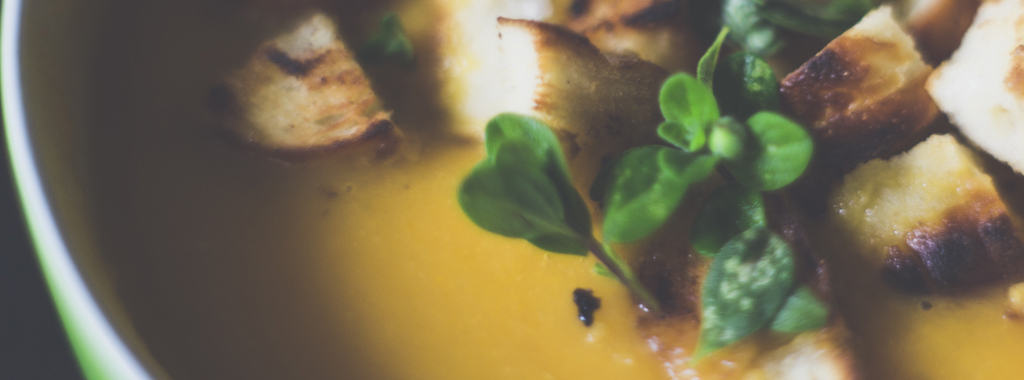
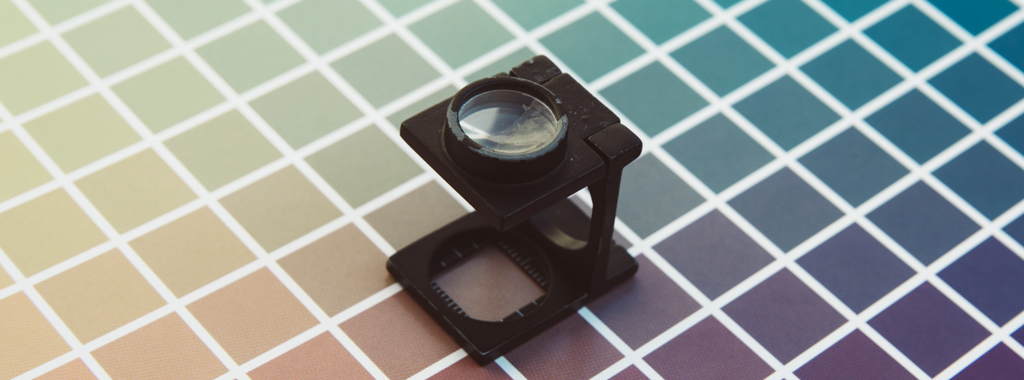
Vertical Carousel
$('#aniCarousel-with-dataattributes').aniCarousel({
vertical: true,
animationIn: 'slideInDown',
animationOut: 'slideOutDown',
nextBtn: '<i class="fa fa-arrow-circle-o-down fa-2x text-primary"></i>',
prevBtn: '<i class="fa fa-arrow-circle-o-up fa-2x text-primary"></i>',
loop: true,
autoplay: true,
overflow: 'hidden',
swipe: true,
paginator: true,
});
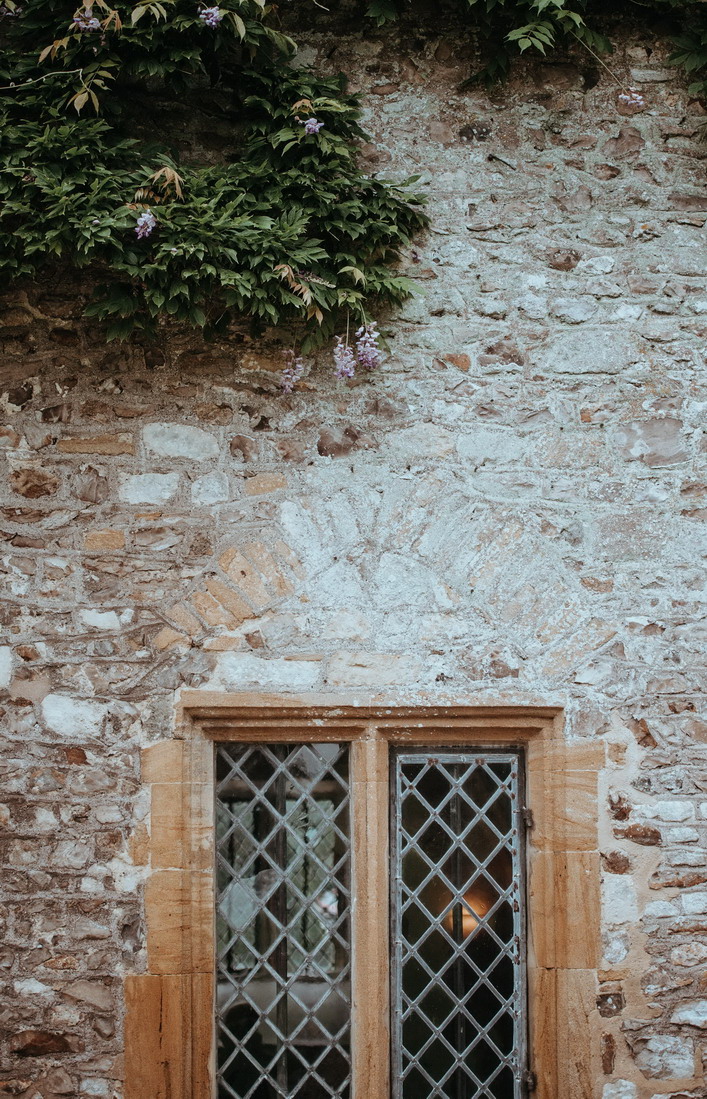
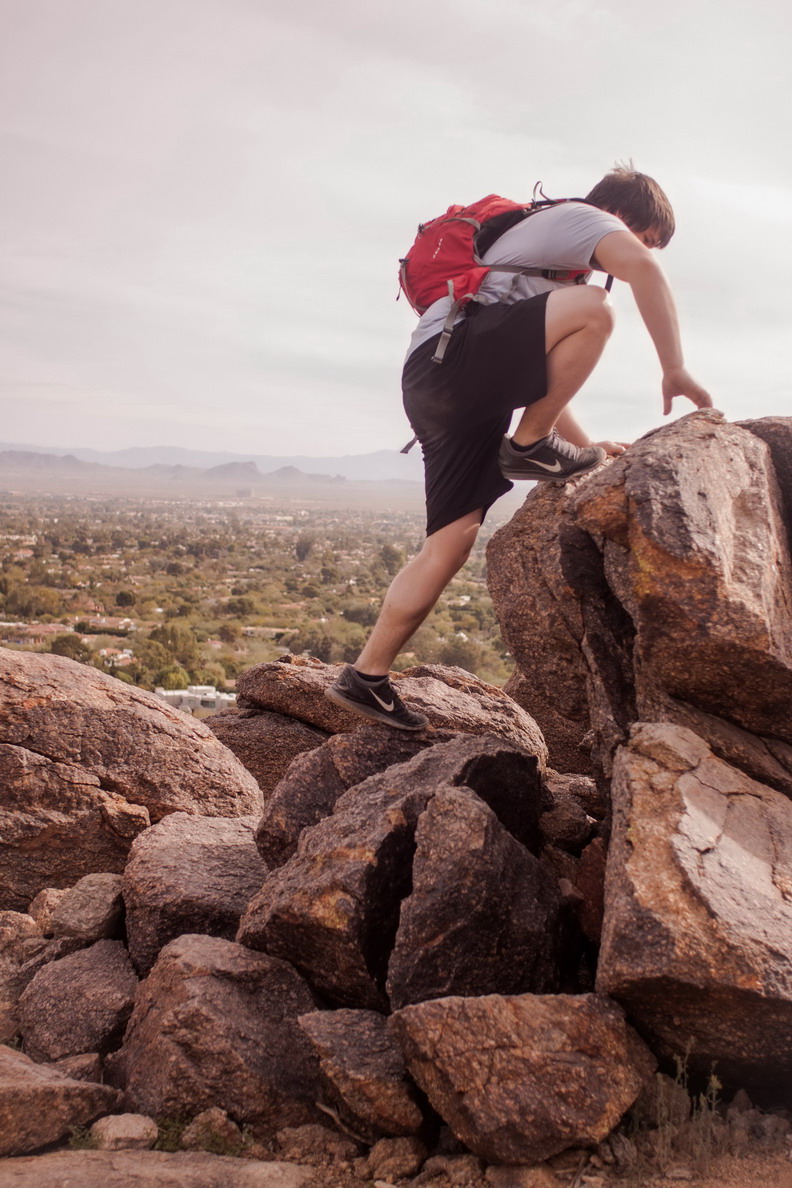
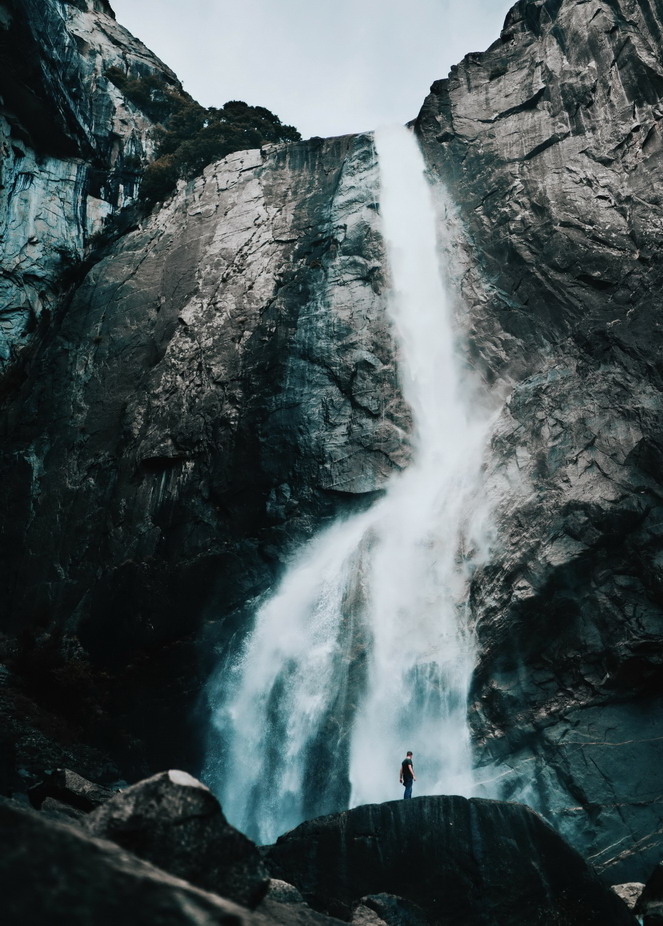
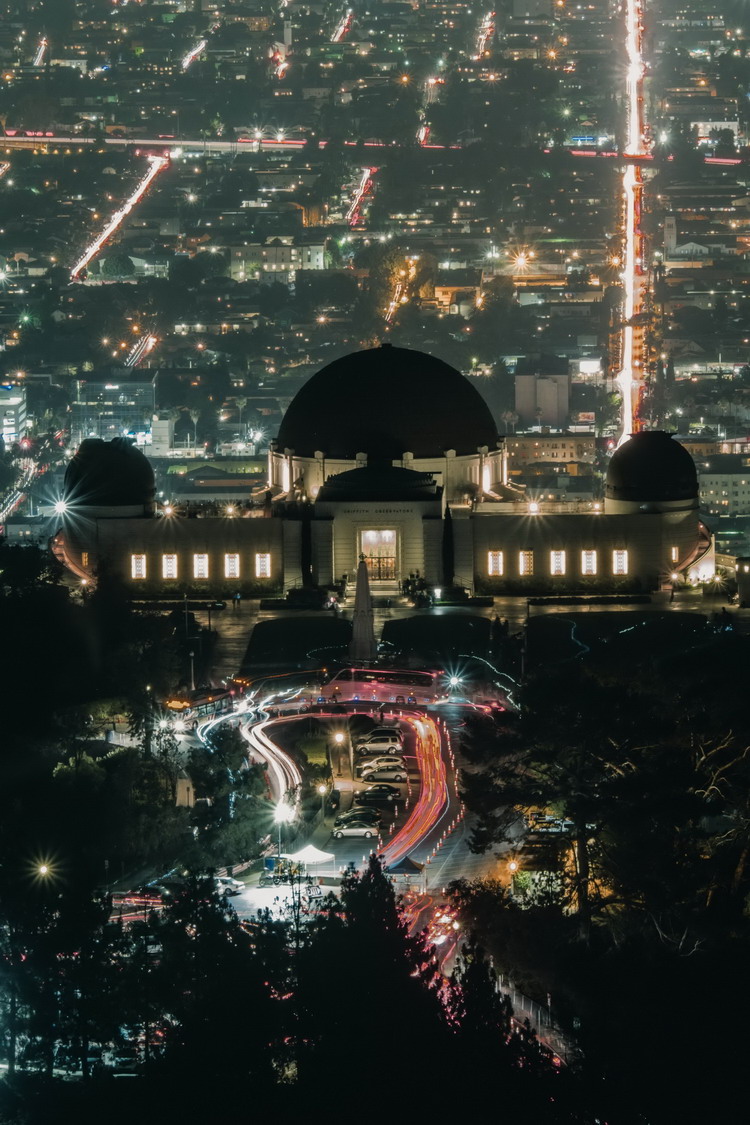
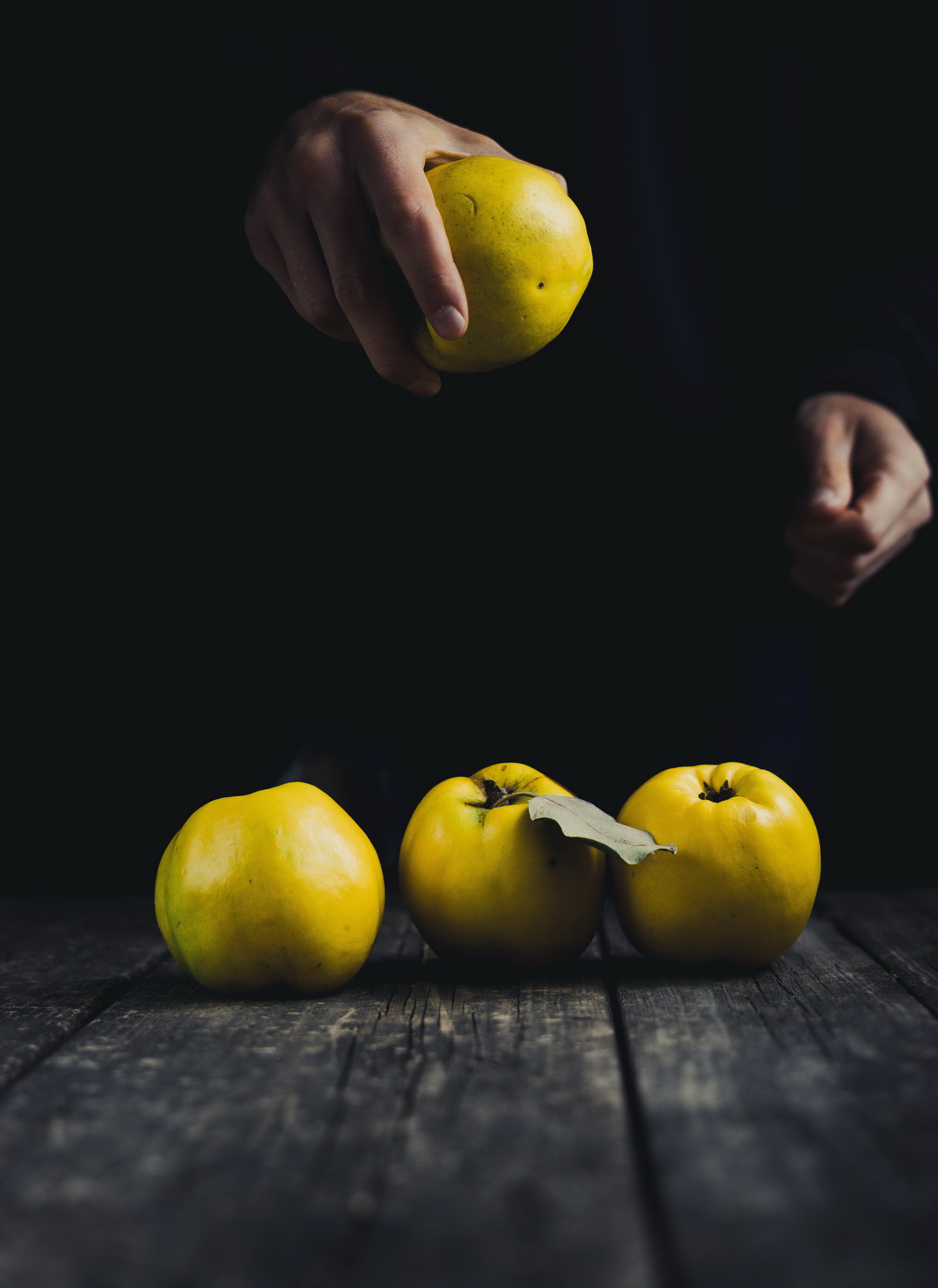
Use of methods
Note: You must init the carousel
$('selector').aniCarousel();
before calling any of the following methods.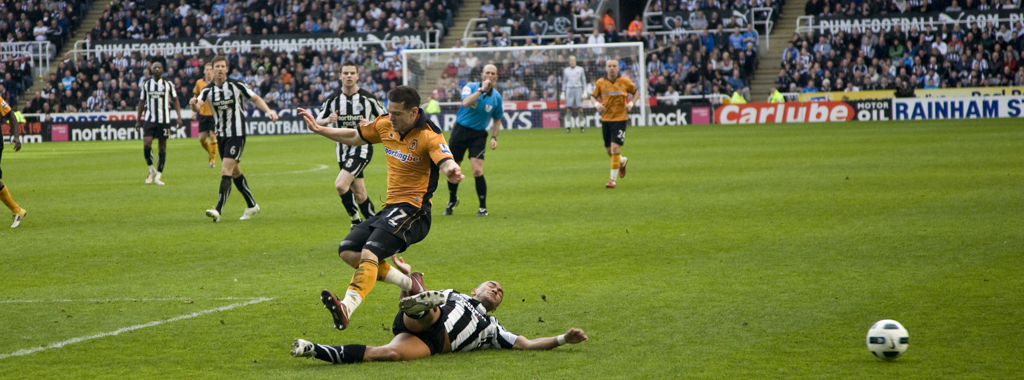
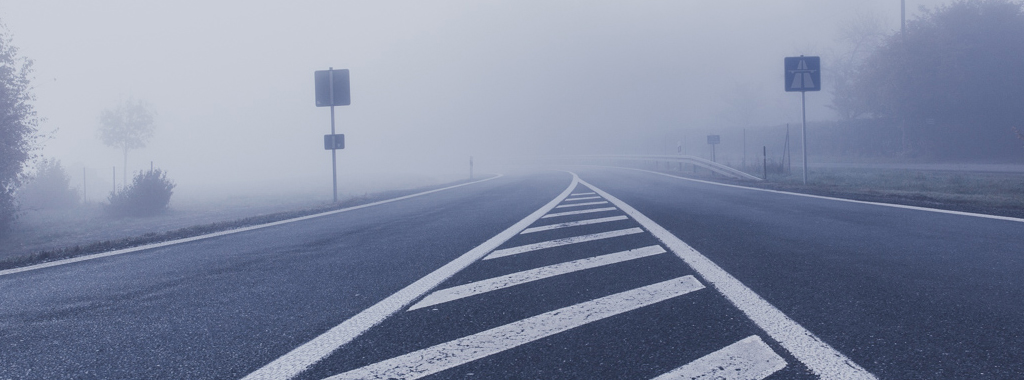
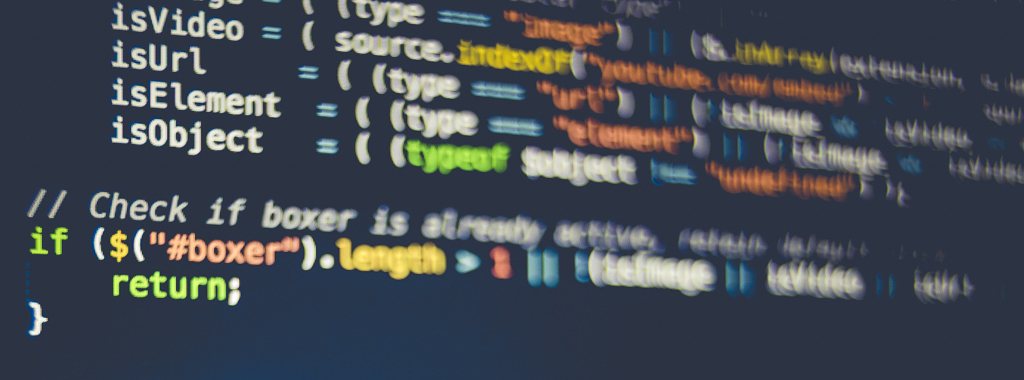
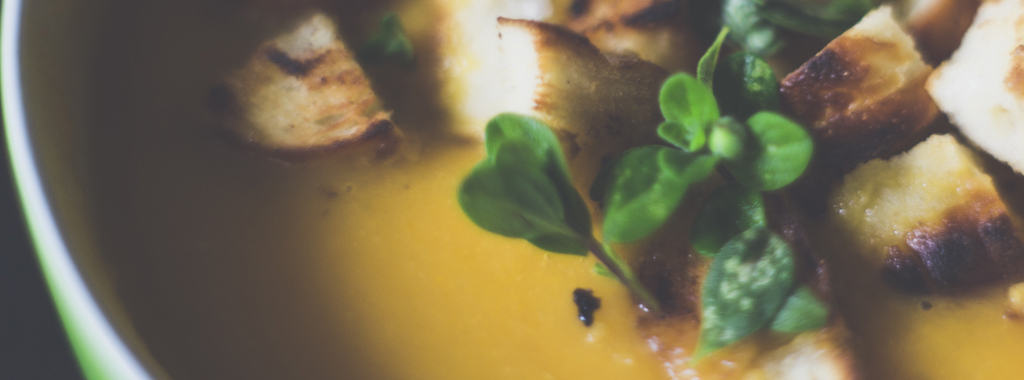
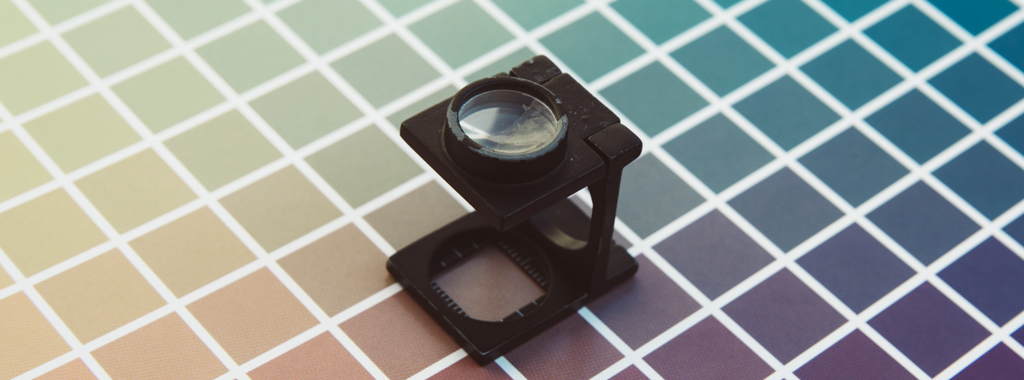
Options and Methods
Available options and methods :
Name | type | default | description |
---|---|---|---|
Options | |||
animator | string | ‘animate’ | CSS Animation library name.
Type false if you aren’t using any Library |
animationIn | string | ‘slideInLeft’ | An animation name from the Animation Library to run when an item/slide going to Show. Wrong name would cause no movement in the carousel. |
animationOut | string | ‘slideOutRight’ | An animation name from the Animation Library to run when an item/slide going to Hide. Wrong name would cause no movement in the carousel. |
autoplay | boolean | false | Whether the carousel should auto slide. |
pauseOnHover | boolean | false | Whether the carousel should stop cycling when mouse/cursor is entered/hovered. |
loop | boolean | false | Whether the carousel should cycle continuously or have hard stops. |
interval | integer | 5 | The amount of time in seconds to delay between automatically cycling an item. |
paginator | boolean | false | Show/Hide dot pagination. |
navigator | boolean | true | Show/Hide Left and Right (or Top and Bottom for vertical) arrow buttons. |
nextBtn | html | Accepts HTML to replace the arrow for Next button. | |
prevBtn | html | AcceptsHTML to replace the arrow for Previous button. | |
swipe | boolean | false | Weather enable/disable the Touch Swipe support. |
vertical | boolean | false | Weather show the carousel as Vertical. |
overflow | string | ” | use hidden if you do not want the animation to be seen outside the container of Carousel. |
afterInit | callback |
afterInit: function(object){} |
Executes right after Carousel is created. Passes object – is carousel itself. |
beforeChange | callback |
beforeChange: function([active_slide, next_slide]){} |
Executes before moving to next slide. Passes array of active_slide – active slide and next_slide – next slide. |
afterChange | callback |
afterChange: function([active_slide]){} |
Executes after moved to next slide. Passes array of active_slide – moved slide. |
Methods Note: You must init the carousel $('selector').aniCarousel(); before calling any of the following methods. |
|||
next | method |
$('#aniCarousel').aniCarousel('next'); |
Moves to next. |
previous | method |
$('#aniCarousel').aniCarousel('previous'); |
Moves to previous. |
to | method |
$('#aniCarousel').aniCarousel('to', integer); |
Moves to the given numbered slide. |
play | method |
$('#aniCarousel').aniCarousel('play'); |
Starts autoplay |
stop | method |
$('#aniCarousel').aniCarousel('stop'); |
Stops autoplay |
destroy | method |
$('#aniCarousel').aniCarousel('destroy'); |
Method to destroy the carousel. |
CSS Style
List of CSS Classes to change styles
.aniCarousel {
}
.aniCarousel-inner {
}
.aniCarousel-item {
}
.aniCarousel-item.active {
}
.aniCarousel-navigator {
}
.aniCarousel-navigator.aniCarousel-prev {
}
.aniCarousel-navigator.aniCarousel-next {
}
.aniCarousel-next-icon,
.aniCarousel-prev-icon {
}
.aniCarousel-next-icon:hover,
.aniCarousel-prev-icon:hover {
}
.aniCarousel-paginator {
}
.aniCarousel-paginator-pager {
}
.aniCarousel-paginator-pager:hover,
.aniCarousel-paginator-pager.selected {
}
.aniCarousel-vertical{
}
.aniCarousel-vertical .aniCarousel-navigator{
}
.aniCarousel-vertical .aniCarousel-navigator.aniCarousel-prev {
}
.aniCarousel-vertical .aniCarousel-navigator.aniCarousel-next {
}
.aniCarousel-vertical .aniCarousel-prev-icon,
.aniCarousel-vertical .aniCarousel-next-icon {
}
.aniCarousel-vertical .aniCarousel-paginator {
}
.aniCarousel-vertical .aniCarousel-paginator-pager {
}